This is part two of a completely unknown number of linked articles as I’m writing them without even the slightest bit of aforethought. You can find the first instalment here. They’re not tutorials as such, but more documenting my progress and thinking as I forge onward building something.
First thing to do is add a property to your User model in Laravel to represent the number of credits a user has. We’ll give a new user 5 credits to start with.
Schema::table('users', function (Blueprint $table) { $table->integer("credit_balance")->default(5); });
Then a method on the User model to add credits to the users balance:
public function addCredits($credits){ $this->credit_balance += $credits; $this->save(); }
Finally, a couple of API endpoints to retrieve the anonymous user’s current balance and top-up the account:
public function balance() { return response()->json(["balance"=>Auth::user()->credit_balance], 200); } public function topUp(Request $request) { $validation = Validator::make($request->all(),[ 'credits' => 'required', ]); if($validation->fails()) return response()->json(["errors"=>$validation->messages()], 200); $user = Auth::user(); $user->addCredits($request->credits); return response()->json(["balance"=>$user->credit_balance], 200); }
Need to get this into the app now, so the user can see their credit balance. For this bit, I’m using Swift UI – if you haven’t played with it yet you ought to, it’s really cool!
I’ve created a BalanceData class which is responsible for loading balance data from our API. This class publishes a balance variable for our views to observe.
import SwiftUI import Alamofire public class BalanceData : ObservableObject { @Published var balance: Int = 0 init(){ updateBalance() } func updateBalance() { let token = NSUbiquitousKeyValueStore.default.string(forKey: "laravelToken") if(token != nil) { let headers: HTTPHeaders = [ "Authorization": "Bearer " + token! ] AF.request("http://credits.test/api/auth/balance",method: .get, encoding:JSONEncoding.default, headers: headers).responseJSON { response in switch response.result { case .success(let JSON): let response = JSON as! NSDictionary DispatchQueue.main.async { self.balance = response["balance"]! as! Int } break case .failure: break } } } } }
My BalanceView file looks like this – nothing fancy, but shows the balance from the server!
import SwiftUI struct BalanceView: View { @EnvironmentObject var balanceData: BalanceData var body: some View { VStack { Text(String(self.balanceData.balance)) .font(.system(size: 72)) .foregroundColor(.accentColor) Text("Credits") .font(.system(size: 18)) .foregroundColor(.gray) } } } struct BalanceView_Previews: PreviewProvider { static var previews: some View { BalanceView() .environmentObject(BalanceData()) } }
Like I said, nothing special but there’s some useful tidbits of code there if you’re thinking of doing something similar.
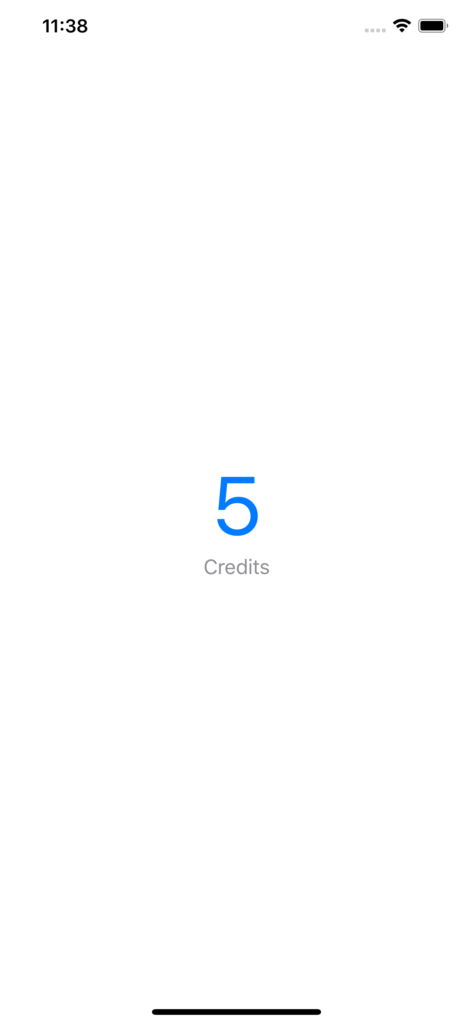
What’s next? Probably updating the users balance from the app.